Streamlining QA Automation with CI/CD Pipelines: Best Practices and Implementation Strategies
Learn how to integrate QA automation into CI/CD pipelines for efficient testing and deployment.
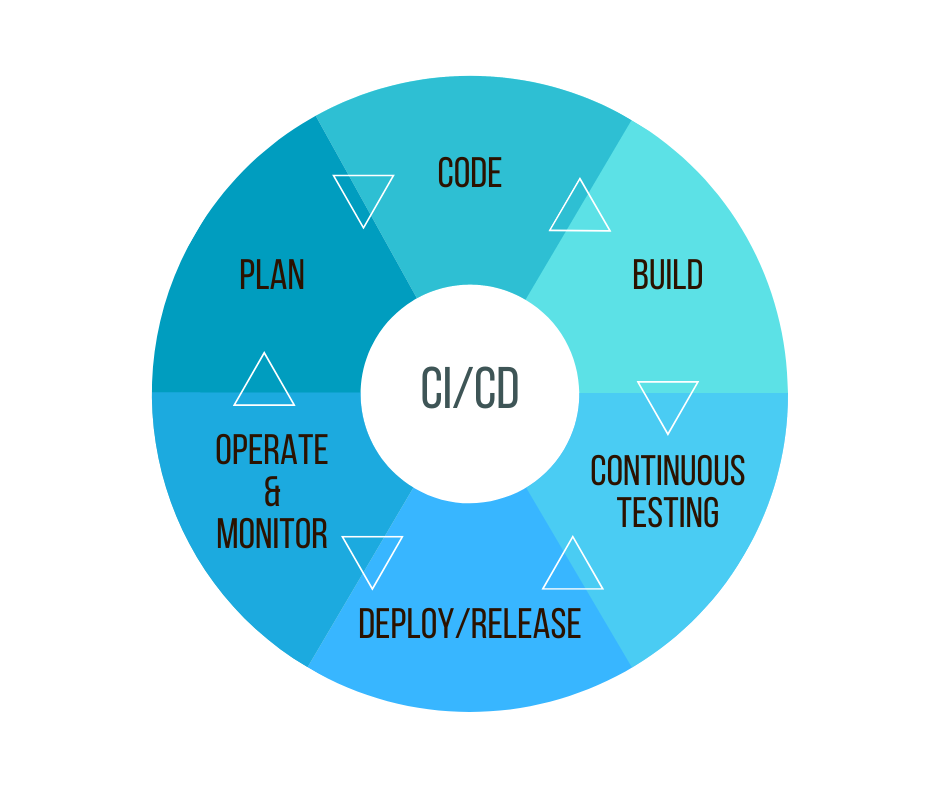
In modern software development, Continuous Integration and Continuous Deployment (CI/CD) pipelines are integral to automating the software delivery process. CI/CD pipelines facilitate the integration of code changes, automated testing, and deployment of applications, enabling teams to deliver high-quality software efficiently and reliably.
Integrating QA automation into CI/CD pipelines offers several benefits:
- Faster Feedback Loops: Automated tests run continuously as part of the pipeline, providing immediate feedback on the quality of code changes. This enables developers to identify and address issues early in the development process, reducing the time and effort required for bug fixes.
- Consistent Testing: Automated tests ensure consistent testing across environments, reducing the risk of human error and ensuring that the application behaves as expected in different scenarios. By automating repetitive testing tasks, QA teams can focus on more complex testing activities that require human intervention.
- Streamlined Deployment: Automated deployment processes enable faster and more reliable releases, with fewer manual interventions. By automating the deployment of applications to various environments, such as staging and production, teams can reduce the risk of deployment errors and ensure consistent deployment practices across environments.
- Improved Collaboration: CI/CD pipelines promote collaboration between development, QA, and operations teams by standardizing workflows and automating repetitive tasks. By automating the integration, testing, and deployment processes, CI/CD pipelines enable teams to work together more efficiently and deliver high-quality software faster.
A typical CI/CD pipeline for QA automation consists of the following components:
-
Source Control: Version control systems such as Git are used to manage the source code and track changes. Developers commit code changes to a central repository, enabling collaboration and versioning of code.
-
Build Automation: Continuous Integration servers like Jenkins, GitHub Actions or GitLab CI automate the build process, compiling code and running unit tests. CI servers monitor the source code repository for changes and trigger builds whenever new code is committed.
-
Automated Testing: QA automation tools execute automated tests, including unit tests, integration tests, and end-to-end tests. These tests verify the functionality and behavior of the application and help ensure its reliability and quality.
-
Deployment Automation: Continuous Deployment tools deploy the application to various environments, such as staging and production, based on predefined criteria. CD tools automate the deployment process, ensuring consistent and reliable deployment practices across environments.
-
Branching Strategy: Implementing a branching strategy such as GitFlow or GitHub Flow is crucial for managing code changes across different environments (e.g., development, QA, staging, production). A well-defined branching strategy helps teams organize and manage code changes effectively, facilitating collaboration and ensuring the stability of each environment.
To ensure the effectiveness and reliability of QA automation in CI/CD pipelines, consider the following best practices:
-
Test Early and Often: Integrate automated tests into the pipeline as early as possible to catch defects sooner in the development process. By running tests continuously throughout the development lifecycle, teams can identify and address issues early, reducing the risk of bugs reaching production.
-
Parallelize Test Execution: Run tests in parallel to reduce overall testing time and increase pipeline efficiency. By parallelizing test execution, teams can accelerate the testing process and obtain faster feedback on the quality of code changes.
-
Use Containers for Isolation: Utilize containerization technologies such as Docker to isolate tests and dependencies, ensuring consistency across environments. Containers provide a lightweight and portable way to package applications and their dependencies, making it easier to manage test environments and dependencies.
-
Monitor Test Results: Implement monitoring and reporting mechanisms to track test results and identify trends or patterns over time. By monitoring test results, teams can identify areas for improvement in test coverage or test reliability and take proactive measures to address issues.
-
Automate Environment Provisioning: Automate the setup and teardown of test environments to ensure consistency and reproducibility. By automating environment provisioning, teams can avoid manual errors and ensure that test environments are configured consistently each time tests are run.
Integrating QA automation tools with CI/CD pipelines requires careful configuration and setup. Here's how you can integrate automation tools into your pipelines:
CLI/API Integration: Many automation tools offer command-line interfaces (CLIs) or APIs that allow you to execute tests within your CI/CD pipeline. By leveraging these interfaces, you can seamlessly integrate automation testing into your existing workflows.
Headless Execution: Configure your automation tests to run in headless mode within CI environments. Headless execution allows tests to run without a graphical user interface, making them suitable for automated CI/CD processes.
Plugin Support: Explore plugin options for your CI/CD tools that facilitate integration with automation frameworks. These plugins can streamline the setup process and provide additional features for managing and executing tests within your pipeline.
Parallel Execution: Utilize techniques such as parallel testing to distribute test execution across multiple nodes or environments. Parallel execution can significantly reduce testing time and improve pipeline efficiency.
Scalability: Consider the scalability of your automation setup, especially if you anticipate a large volume of tests or concurrent executions. Ensure that your automation solution can handle increased workload demands without compromising performance.
Reporting and Monitoring: Implement robust reporting and monitoring mechanisms to track test results, identify failures, and analyze trends. Real-time visibility into test execution status helps teams quickly identify issues and take corrective actions as needed.
Environment Configuration: Automate the setup and teardown of test environments to ensure consistency and reproducibility across different stages of your CI/CD pipeline. Environment configuration automation reduces manual errors and ensures reliable test execution.
By following these guidelines, you can effectively integrate QA automation into your CI/CD pipelines, improving software quality, accelerating release cycles, and enhancing overall development efficiency.
# Official framework image. Look for the different tagged releases at:
# https://hub.docker.com/r/library/node/tags/
image: node:latest
# Pick zero or more services to be used on all builds.
# Only needed when using a docker container to run your tests in.
services:
- mysql:latest
- redis:latest
- postgres:latest
# This folder is cached between builds
cache:
paths:
- node_modules/
test_async:
script:
- npm install
- node ./specs/start.js ./specs/async.spec.js
test_db:
script:
- npm install
- node ./specs/start.js ./specs/database.spec.js
deploy:
stage: deploy
script: echo "Define your deployment script!"
environment: production
For more details and examples, check out the official GitLab documentation.
# Workflow name
name: Node.js CI
# List of events you want to trigger the workflow
on: [push]
# List of jobs
jobs:
build:
runs-on: ubuntu-latest
# List of steps
steps:
# Pull the source code
- name: Checkout
uses: actions/checkout@v4
# Setup Node.js
- name: Use Node.js
uses: actions/setup-node@v4
with:
node-version: "20.x"
# Install all required dependencies
- name: Install dependencies
run: npm ci
# Build
- name: Build
run: npm run build --if-present
# Run tests
- name: Run tests
run: npm test
For more details and examples, check out the official GitHub documentation.
pipeline {
agent none
stages {
stage('Build') {
agent any
steps {
checkout scm
sh 'make'
stash includes: '**/target/*.jar', name: 'app'
}
}
stage('Test on Linux') {
agent {
label 'linux'
}
steps {
unstash 'app'
sh 'make check'
}
post {
always {
junit '**/target/*.xml'
}
}
}
stage('Test on Windows') {
agent {
label 'windows'
}
steps {
unstash 'app'
bat 'make check'
}
post {
always {
junit '**/target/*.xml'
}
}
}
}
}
For more details and examples, check out the official Jenkins documentation.
Continuous monitoring is essential for ensuring the reliability and effectiveness of CI/CD pipelines for QA automation. Implement monitoring tools and practices to:
- Track pipeline performance metrics such as build times, test execution times, and failure rates. By monitoring these metrics, teams can identify bottlenecks and areas for improvement in the pipeline.
- Receive alerts and notifications for failed tests or pipeline issues. By configuring alerts and notifications, teams can respond quickly to failures and take corrective actions to ensure the stability of the pipeline.
- Analyze test results and identify areas for improvement in test coverage or test reliability. By analyzing test results, teams can identify trends or patterns in test failures and take proactive measures to address underlying issues.
By integrating QA automation into CI/CD pipelines, teams can streamline their testing and deployment processes, accelerate time-to-market, and improve overall software quality. By following best practices, leveraging appropriate tooling, embracing a culture of continuous improvement, and adopting a robust branching strategy, organizations can achieve greater efficiency and reliability in their software delivery lifecycle.