Comprehensive Guide to CI/CD with GitHub Actions: Best Practices and Examples
Learn GitHub Actions for testing with efficient CI/CD pipeline implementation in software development.
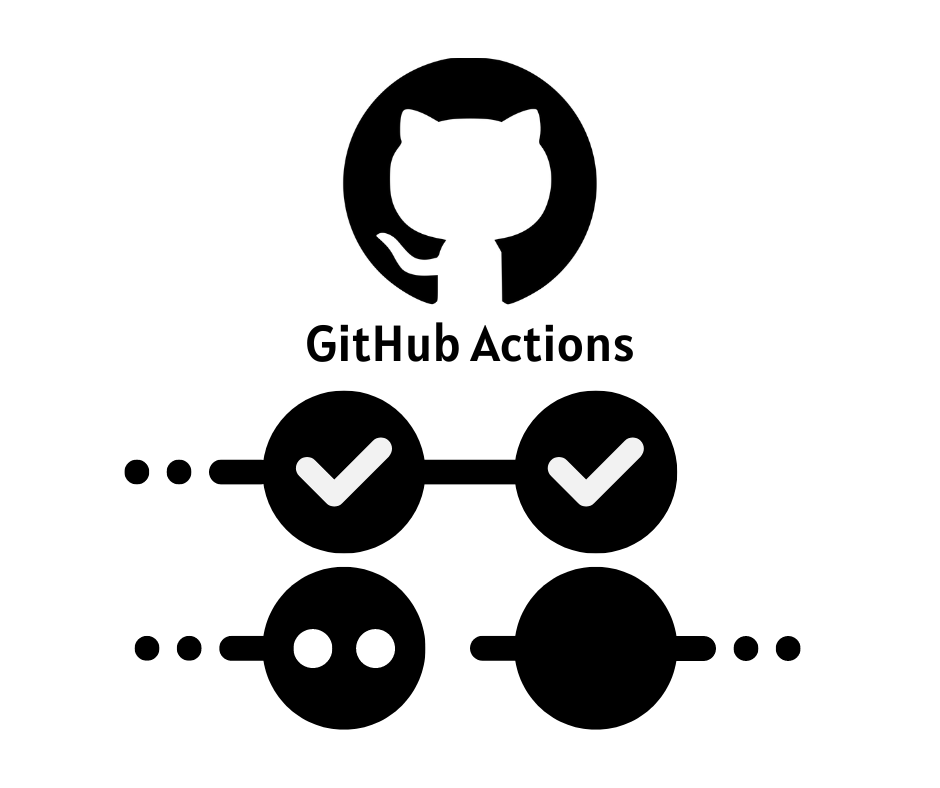
GitHub Actions is a powerful tool provided by GitHub for automating workflows directly within your GitHub repository. With GitHub Actions, you can build, test, and deploy your code right from your repository, enabling efficient Continuous Integration and Continuous Deployment (CI/CD) practices. In this guide, we'll explore best practices and provide examples to help you implement CI/CD pipelines using GitHub Actions effectively.
GitHub Actions offers several benefits for implementing CI/CD pipelines:
Native Integration: GitHub Actions is tightly integrated with GitHub repositories, allowing seamless automation of workflows directly within your development environment.
Versatility: GitHub Actions supports a wide range of programming languages, platforms, and deployment targets, providing flexibility for diverse development environments.
Workflow Customization: You can customize workflows to suit your specific requirements, including building, testing, and deploying applications in different environments.
Scalability: GitHub Actions scales effortlessly with your projects, accommodating small teams and large enterprises alike, without requiring additional infrastructure setup.
Community Support: GitHub Actions has a vibrant community contributing workflows, actions, and best practices, enabling collaborative development and sharing of automation solutions.
To begin using GitHub Actions for CI/CD, follow these steps:
Create a Workflow File: Create a .github/workflows directory in your repository and add YAML workflow files defining your CI/CD pipelines. Each workflow file represents a set of actions to be executed based on specific triggers.
Define Workflow Triggers: Specify triggers for your workflows, such as on push events, pull requests, or on a schedule. Workflows can also be triggered manually or based on external events.
Configure Workflow Steps: Define the steps to be executed in your workflows. Steps can include checking out code, running tests, building artifacts, and deploying applications.
Commit and Push: Commit your workflow files to your repository and push them to trigger the workflows. GitHub Actions will automatically execute the defined workflows based on the specified triggers.
To ensure efficient and reliable CI/CD pipelines with GitHub Actions, consider the following best practices:
Modular Workflows: Break down workflows into smaller, reusable components called actions. Use action marketplace or create custom actions to encapsulate common tasks and promote code reuse.
Containerization: Utilize Docker containers for building and testing applications within workflows. Containerization ensures consistency across environments and simplifies dependency management.
Secret Management: Safeguard sensitive information such as API tokens, credentials, and deployment keys using GitHub Secrets. Avoid hardcoding secrets in workflow files and utilize encrypted secrets for secure access.
Artifact Management: Archive build artifacts or generated files as workflow artifacts for traceability and reproducibility. Use the actions/upload-artifact and actions/download-artifact actions to store and retrieve artifacts between workflow steps.
Parallelism: Parallelize workflow steps to reduce overall execution time. Use the jobs.<job_id>.strategy.matrix feature to run tests or tasks concurrently across different environments or configurations.
Here's an example workflow for a Node.js application demonstrating CI/CD with GitHub Actions:
name: Your Workflow Name DEV
on:
workflow_run:
workflows: [Your Deploy workflow DEV]
types:
- completed
workflow_dispatch:
concurrency:
group: ${{ github.head_ref || github.ref_name }}
cancel-in-progress: false
Explanation:
-
name: of the workflow/pipeline should always tell what the workflow should achieve, and specifically for automated tests, also which environment it is targeting, e.g., DEV, QA, etc. You can include that info in the name (e.g., Your Workflow Name DEV).
-
on: is used to select event triggers. In this case, we want to run the automated tests on the DEV environment after the application was deployed to DEV (completed). We do that by putting the name of the deploy workflow in the list (on.workflow_run.workflows:). Also, you can add the environment name that the deploy is targeting, so you can easily distinguish which workflow you need to wait for deployment.
on.workflow_dispatch: allows you to trigger the workflow manually from any branch you select from the dropdown in the GitHub UI
-
concurrency: is not necessary but a good practice to separate runs per branch/ref name. This way, the newly triggered run will wait for the pending run.
Next section is usually defining environment variables:
env:
BASE_URL: ${{ vars.BASE_URL }}
SECRET: ${{ secrets.YOUR_SECRET }}
# Other environment variables/secrets
Explanation:
In this section, define all of the needed variables/secrets your test automation framework needs in order to run. Each of them should be added in the GitHub repository Settings. In order to add required variables/secrets that you need to pull into your workflow, you will need admin access to the repository. If you are the owner of the repository, you should already have the access.
The next section is a job itself (or multiple jobs) that will do the magic of running the wanted tests and configuration on the CI/CD:
jobs:
your-job-name:
timeout-minutes: 60
runs-on: ubuntu-latest
steps:
- name: Checkout
uses: actions/checkout@v4
with:
ref: dev
- name: Setup Node
uses: actions/setup-node@v4
with:
node-version: "18.16"
# or any other runtime environment
- name: Install dependencies
run: |
# Commands to install your dependencies
- name: Run tests
run: |
# Commands to run your tests
- name: Upload Artifact
uses: actions/upload-artifact@v4
if: always() # Run even if tests fail
with:
name: name-of-generated-artifact
path: |
# Path to the generated artifact
retention-days: 30 # How many days you want GitHub to store your test results
- name: Test Summary
if: always() # Run even if tests fail
uses: test-summary/action@v2
with:
paths: path-to-your-test-results
# Add any additional post-run actions here, such as sending emails/notifications, etc.
Explanation:
In this part of the workflow, we have defined a job with a few actions involved:
- Checks out the code from the specified branch (dev)
- Sets up Node.js (you should set up all required tools before running your automated tests)
- Installs dependencies
- Runs tests
- After running tests, the workflow uploads generated artifacts and provides a test summary. You can add any additional post-run actions here, such as sending emails/notifications.
In case you have more test suites (UI, API, multiple browser configurations, etc.), and you want to speed up the test execution, you can parallelize using the strategy.matrix feature:
parallel-jobs:
timeout-minutes: 60
runs-on: ubuntu-latest
strategy:
fail-fast: false
matrix:
browser: [msedge, chrome]
steps:
- name: Checkout
uses: actions/checkout@v4
with:
ref: dev
- name: Setup Node
uses: actions/setup-node@v4
with:
node-version: "18.16"
# or any other runtime environment
- name: Install dependencies
run: |
# Commands to install your dependencies
- name: Run tests
run: |
# Commands to run your tests using matrix
npm run test:{{ matrix.browser }}
Explanation:
The strategy.matrix section defines the custom list of configurations that we will use for our tests. Also, it will use these names for creating multiple jobs that will run in parallel.
In this case, fail-fast is set to false, meaning all configurations will run even if one fails.
GitHub Actions provides a robust platform for implementing CI/CD pipelines seamlessly integrated with your GitHub repositories. By following best practices and leveraging GitHub Actions' powerful features, you can automate your software development lifecycle, accelerate release cycles, and improve overall development efficiency. Start exploring GitHub Actions today and unleash the full potential of automated workflows for your projects.